일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- DFS
- GT-S80
- index
- imread
- RNN
- LSTM
- Numpy
- 알고리즘
- Lotto
- javascript
- keras
- CNN
- Python
- GitHub
- pandas
- synology
- ipad
- Series
- install
- mariadb
- mean
- SciPy
- SPL
- pycharm
- E-P1
- Splunk
- pip
- 삼성소프트웨어멤버십
- Button
- dataframe
- Today
- Total
잠토의 잠망경
학교 강의 내용 2st 본문
Date.cs
===================================================================================
using System;
class Date
{
int year = 1;
int month = 1;
int day = 1;
public int Year
{
set
{/**************************************
* Property의 사용법
* 배울 점 1
* ************************************/
if (!IsConsistent(value, Month, Day))
throw new ArgumentOutOfRangeException("Year");
year = value;
}
get
{ return year; }
}
public int Month
{
set
{
if (!IsConsistent(Year, value, Day))
throw new ArgumentOutOfRangeException("Year");
month = value;
}
get { return month; }
}
public int Day
{
set
{
if (!IsConsistent(Year, Month, value))
throw new ArgumentOutOfRangeException("Year");
day = value;
}
get { return day; }
}
public Date() { }
public Date(int year, int month, int day)
{
Year = year;
Month = month;
Day = day;
}
static bool IsConsistent(int year, int month, int day)
{
if (year < 1)
return false;
if (month < 1 || month > 12)
return false;
if (day < 1 || day > 31)
return false;
if (day == 31 && (month == 4 || month == 6 ||
month == 9 || month == 11))
return false;
if (month == 2 && day > 29)
return false;
if (month == 2 && day == 29 && IsLeapYear(year))
return false;
return true;
}
/***********************************
*
* 윤년 계산법
*
* *********************************/
public static bool IsLeapYear(int year)
{
return year % 4 == 0 && (year % 100 != 0 || year % 400 == 0);
}
/***********************************
*
* 누적 날수
*
* ********************************/
static int[] daysCumulative ={ 0, 31, 59, 90, 120, 151, 181, 212, 243, 273, 304, 334 };
public int DayOfYear()
{
return daysCumulative[Month - 1] + Day + (Month > 2 && IsLeapYear(Year) ? 1 : 0);
}
static string[] strMonths = {"Jan", "Feb","Mar","Apr","May", "Jun", "Jul", "Aug", "Sep",
"Oct", "Nov", "Dec"};
public override string ToString()
{
return String.Format("{0} {1} {2}", Day, strMonths[Month - 1], Year);
}
}
===================================================================================
Inheritance.cs
===================================================================================
using System;
class ExtendedDate : Date
{
public ExtendedDate() {}
public ExtendedDate(int iYear, int iMonth, int iDay)
:base(iYear, iMonth, iDay)
{
}
}
class Inheritance
{
static void Main()
{
ExtendedDate dateMoonWalk = new ExtendedDate(1969, 7, 20);
Console.WriteLine("Moon walk: {0}, Day of Year: {1}", dateMoonWalk,
dateMoonWalk.DayOfYear());
}
}
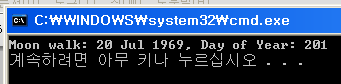